
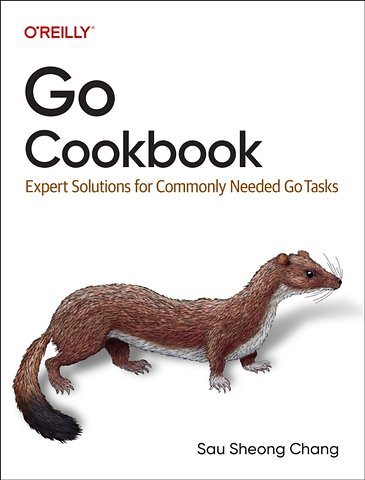
I am currently the Director of the Applied Cloud Computing Lab in HP Labs Singapore, responsible for managing a team of engineers focusing on researching, developing and testing cloud computing solutions.
Meer over Sau Sheong ChangGo Cookbook
Expert Solutions for Commonly Needed Go Tasks
Paperback Engels 2023 1e druk 9781098122119Samenvatting
Go is an increasingly popular language for programming everything from web applications to distributed network services. This practical guide provides recipes to help you unravel common problems and perform useful tasks when working with Go. Each recipe includes self-contained code solutions that you can freely use, along with a discussion of how and why they work. Programmers new to Go can quickly ramp up their knowledge while accomplishing useful tasks, and experienced Go developers can save time by cutting and pasting proven code directly into their applications.
Recipes include:
- Creating a module
- Calling code from another module
- Converting strings to numbers (or converting numbers to strings)
- Modifying multiple characters in a string
- Creating substrings from a string
- Capturing string input
- And so much more
Specificaties
Lezersrecensies
Inhoudsopgave
Conventions Used in This Book
Using Code Examples
O’Reilly Online Learning
How to Contact Us
Acknowledgments
1. Getting Started Recipes
1.0. Introduction
1.1. Installing Go
1.2. Playing Around with Go
1.3. Writing a Hello World Program
1.4. Using an External Package
1.5. Handling Errors
1.6. Logging Events
1.7. Testing Your Code
2. Module Recipes
2.0. Introduction
2.1. Creating a Go Module
2.2. Importing Dependent Packages Into Your Module
2.3. Removing Dependent Packages from Your Module
2.4. Find Available Versions of Third-Party Packages
2.5. Importing a Specific Version of a Dependent Package Into Your Module
2.6. Requiring Local Versions of Dependent Packages
2.7. Using Multiple Versions of the Same Dependent Packages
3. Error Handling Recipes
3.0. Introduction
3.1. Handling Errors
3.2. Simplifying Repetitive Error Handling
3.3. Creating Customized Errors
3.4. Wrapping an Error with Other Errors
3.5. Inspecting Errors
3.6. Handling Errors with Panic
3.7. Recovering from Panic
3.8. Handling Interrupts
4. Logging Recipes
4.0. Introduction
4.1. Writing to Logs
4.2. Change What Is Being Logged by the Standard Logger
4.3. Logging to File
4.4. Using Log Levels
4.5. Logging to the System Log Service
5. Function Recipes
5.0. Introduction
5.1. Defining a Function
5.2. Accepting Multiple Data Types with a Function
5.3. Accepting a Variable Number of Parameters
5.4. Accepting Parameters of Any Type
5.5. Creating an Anonymous Function
5.6. Creating a Function That Maintains State After It Is Called
6. String Recipes
6.0. Introduction
6.1. Creating Strings
6.2. Converting String to Bytes and Bytes to String
6.3. Creating Strings from Other Strings and Data
6.4. Converting Strings to Numbers
6.5. Converting Numbers to Strings
6.6. Replacing Multiple Characters in a String
6.7. Creating a Substring from a String
6.8. Checking if a String Contains Another String
6.9. Splitting a String Into an Array of Strings or Combining an Array of Strings Into a String
6.10. Trimming Strings
6.11. Capturing String Input from the Command Line
6.12. Escaping and Unescaping HTML Strings
6.13. Using Regular Expressions
7. General Input/Output Recipes
7.0. Introduction
7.1. Reading from an Input
7.2. Writing to an Output
7.3. Copying from a Reader to a Writer
7.4. Reading from a Text File
7.5. Writing to a Text File
7.6. Using a Temporary File
8. CSV Recipes
8.0. Introduction
8.1. Reading the Whole CSV File
8.2. Reading a CSV File One Row at a Time
8.3. Unmarshalling CSV Data Into Structs
8.4. Removing the Header Line
8.5. Using Different Delimiters
8.6. Ignoring Rows
8.7. Writing CSV Files
8.8. Writing to File One Row at a Time
9. JSON Recipes
9.0. Introduction
9.1. Parsing JSON Data Byte Arrays to Structs
9.2. Parsing Unstructured JSON Data
9.3. Parsing JSON Data Streams Into Structs
9.4. Creating JSON Data Byte Arrays from Structs
9.5. Creating JSON Data Streams from Structs
9.6. Omitting Fields in Structs
10. Binary Recipes
10.0. Introduction
10.1. Encoding Data to gob Format Data
10.2. Decoding gob Format Data to Structs
10.3. Encoding Data to a Customized Binary Format
10.4. Decoding Data with a Customized Binary Format to Structs
11. Date and Time Recipes
11.0. Introduction
11.1. Telling Time
11.2. Doing Arithmetic with Time
11.3. Representing Dates
11.4. Representing Time Zones
11.5. Representing Duration
11.6. Pausing for a Specific Duration
11.7. Measuring Lapsed Time
11.8. Formatting Time for Display
11.9. Parsing Time Displays Into Structs
12. Structs Recipes
12.0. Introduction
12.1. Defining Structs
12.2. Creating Struct Methods
12.3. Creating and Using Interfaces
12.4. Creating Struct Instances
12.5. Creating One-Time Structs
12.6. Composing Structs from Other Structs
12.7. Defining Metadata for Struct Fields
13. Data Structure Recipes
13.0. Introduction
13.1. Creating Arrays or Slices
13.2. Accessing Arrays or Slices
13.3. Modifying Arrays or Slices
13.4. Making Arrays and Slices Safe for Concurrent Use
13.5. Sorting Arrays of Slices
13.6. Creating Maps
13.7. Accessing Maps
13.8. Modifying Maps
13.9. Sorting Maps
14. More Data Structure Recipes
14.0. Introduction
14.1. Creating Queues
14.2. Creating Stacks
14.3. Creating Sets
14.4. Creating Linked Lists
14.5. Creating Heaps
14.6. Creating Graphs
14.7. Finding the Shortest Path on a Graph
15. Image-Processing Recipes
15.0. Introduction
15.1. Loading an Image from a File
15.2. Saving an Image to a File
15.3. Creating Images
15.4. Flipping an Image Upside Down
15.5. Converting an Image to Grayscale
15.6. Resizing an Image
16. Networking Recipes
16.0. Introduction
16.1. Creating a TCP Server
16.2. Creating a TCP Client
16.3. Creating a UDP Server
16.4. Creating a UDP Client
17. Web Recipes
17.0. Introduction
17.1. Creating a Simple Web Application
17.2. Handling HTTP Requests
17.3. Handling HTML Forms
17.4. Uploading a File to a Web Application
17.5. Serving Static Files
17.6. Creating a JSON Web Service API
17.7. Serving Through HTTPS
17.8. Using Templates for Go Web Applications
17.9. Making an HTTP Client Request
18. Testing Recipes
18.0. Introduction
18.1. Automating Functional Tests
18.2. Running Multiple Test Cases
18.3. Setting Up and Tearing Down Before and After Tests
18.4. Creating Subtests to Have Finer Control Over Groups of Test Cases
18.5. Running Tests in Parallel
18.6. Generating Random Test Inputs for Tests
18.7. Measuring Test Coverage
18.8. Testing a Web Application or a Web Service
19. Benchmarking Recipes
19.0. Introduction
19.1. Automating Performance Tests
19.2. Running Only Performance Tests
19.3. Avoiding Test Fixtures in Performance Tests
19.4. Changing the Timing for Running Performance Tests
19.5. Running Multiple Performance Test Cases
19.6. Comparing Performance Test Results
19.7. Profiling a Program
Index
About the Author
Anderen die dit boek kochten, kochten ook
Rubrieken
- advisering
- algemeen management
- coaching en trainen
- communicatie en media
- economie
- financieel management
- inkoop en logistiek
- internet en social media
- it-management / ict
- juridisch
- leiderschap
- marketing
- mens en maatschappij
- non-profit
- ondernemen
- organisatiekunde
- personal finance
- personeelsmanagement
- persoonlijke effectiviteit
- projectmanagement
- psychologie
- reclame en verkoop
- strategisch management
- verandermanagement
- werk en loopbaan