
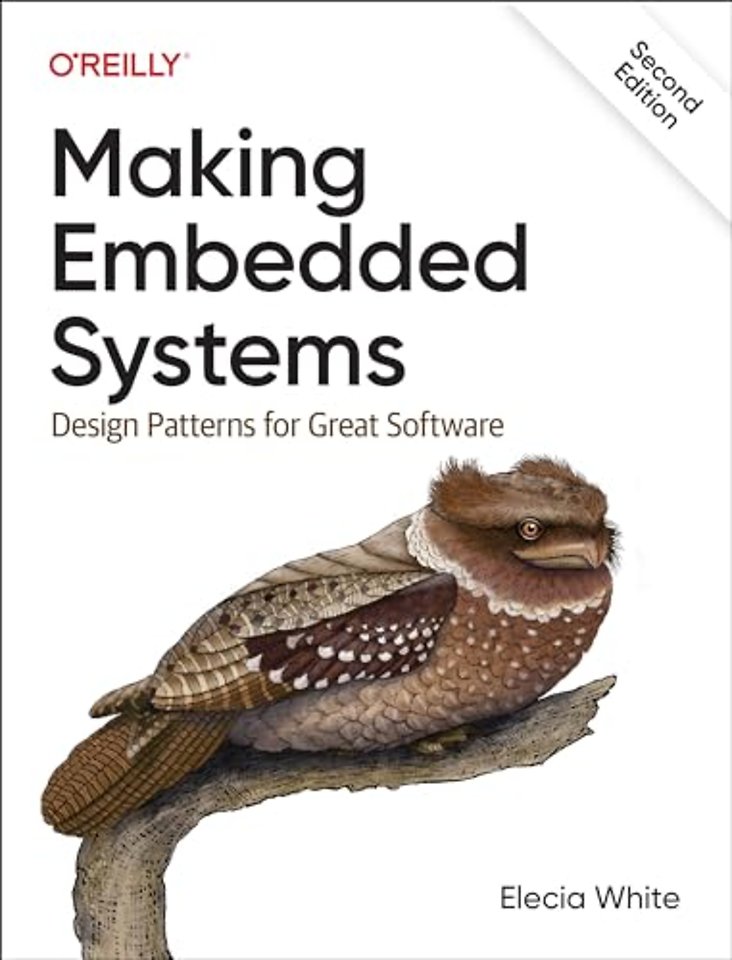
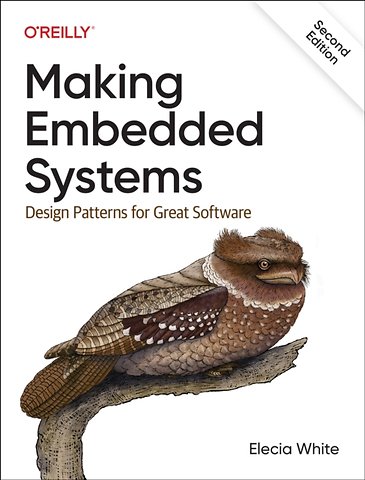
Elecia White has worked on DNA scanners, inertial measurement units for airplanes and race cars, toys for preschoolers, a gunshot location system for catching criminals, and assorted other medical and consumer devices.
Meer over Elecia WhiteMaking Embedded Systems
Design Patterns for Great Software
Paperback Engels 2024 2e druk 9781098151546Samenvatting
Interested in developing embedded systems? Since they don't tolerate inefficiency, these systems require a disciplined approach to programming. This easy-to-read guide helps you cultivate good development practices based on classic software design patterns and new patterns unique to embedded programming. You'll learn how to build system architecture for processors, not for operating systems, and you'll discover techniques for dealing with hardware difficulties, changing designs, and manufacturing requirements.
Written by an expert who has created systems ranging from DNA scanners to children's toys, this book is ideal for intermediate and experienced programmers, no matter what platform you use. This expanded second edition includes new chapters on IoT and networked sensors, motors and movement, debugging, data handling strategies, and more.
- Optimize your system to reduce cost and increase performance
- Develop an architecture that makes your software robust in resource-constrained environments
- Explore sensors, displays, motors, and other I/O devices
- Reduce RAM and power consumption, code space, and processor cycles
- Learn how to interpret schematics, datasheets, and power requirements
- Discover how to implement complex mathematics and machine learning on small processors
- Design effective embedded systems for IoT and networked sensors
Specificaties
Lezersrecensies
Inhoudsopgave
About This Book
Who This Book Is For
About the Author
Organization of This Book
Terminology
Conventions Used in This Book
Using Code Examples
O’Reilly Online Learning
How to Contact Us
Acknowledgments
1. Introduction
Embedded Systems Development
Compilers and Languages
Debugging
Resource Constraints
Principles to Confront Those Challenges
Prototypes and Maker Boards
Further Reading
2. Creating a System Architecture
Getting Started
Creating System Diagrams
The Context Diagram
The Block Diagram
Organigram
Layering Diagram
Designing for Change
Encapsulate Modules
Delegation of Tasks
Driver Interface: Open, Close, Read, Write, IOCTL
Adapter Pattern
Creating Interfaces
Example: A Logging Interface
A Sandbox to Play In
Back to the Drawing Board
Further Reading
3. Getting Your Hands on the Hardware
Hardware/Software Integration
Ideal Project Flow
Hardware Design
Board Bring-Up
Reading a Datasheet
Datasheet Sections You Need When Things Go Wrong
Datasheet Sections for Software Developers
Evaluating Components Using the Datasheet
Your Processor Is a Language
Reading a Schematic
Practice Reading a Schematic: Arduino!
Keep Your Board Safe
Creating Your Own Debugging Toolbox
Digital Multimeter
Oscilloscopes and Logic Analyzers
Setting Up a Scope
Testing the Hardware (and Software)
Building Tests
Flash Test Example
Command and Response
Command Pattern
Dealing with Errors
Consistent Methodology
Error Checking Flow
Error-Handling Library
Debugging Timing Errors
Further Reading
4. Inputs, Outputs, and Timers
Handling Registers
Binary and Hexadecimal Math
Bitwise Operations
Test, Set, Clear, and Toggle
Toggling an Output
Setting the Pin to Be an Output
Turning On the LED
Blinking the LED
Troubleshooting
Separating the Hardware from the Action
Board-Specific Header File
I/O-Handling Code
Main Loop
Facade Pattern
The Input in I/O
Momentary Button Press
Interrupt on a Button Press
Configuring the Interrupt
Debouncing Switches
Runtime Uncertainty
Increasing Code Flexibility
Dependency Injection
Using a Timer
Timer Pieces
Doing the Math
More Math: Difficult Goal Frequency
A Long Wait Between Timer Ticks
Using a Timer
Using Pulse-Width Modulation
Shipping the Product
Further Reading
5. Interrupts
A Chicken Presses a Button
An IRQ Happens
Nonmaskable Interrupts
Interrupt Priority
Nested Interrupts
Save the Context
Retrieve the ISR from the Vector Table
Initializing the Vector Table
Looking Up the ISR
Call the ISR
Multiple Sources for One Interrupt
Disabling Interrupts
Critical Sections
Restore the Context
Configuring Interrupts
When and When Not to Use Interrupts
How to Avoid Using Interrupts
Polling
System Tick
Time-Based Events
A Very Small Scheduler
Further Reading
6. Managing the Flow of Activity
Scheduling and Operating System Basics
Tasks
Communication Between Tasks
Avoiding Race Conditions
Priority Inversion
State Machines
State Machine Example: Stoplight Controller
State-Centric State Machine
State-Centric State Machine with Hidden Transitions
Event-Centric State Machine
State Pattern
Table-Driven State Machine
Choosing a State Machine Implementation
Watchdog
Main Loops
Polling and Waiting
Timer Interrupt
Interrupts Do Everything
Interrupts Cause Events
Very Small Scheduler
Active Objects
Further Reading
7. Communicating with Peripherals
Serial Communication
TTL Serial
RS-232 Serial
SPI
I2C and TWI
1-Wire
Parallel
Dual and Quad SPI
USB
Considering Other Protocols
Communications in Practice
External ADC Example: Data Ready with SPI
Use a FIFO If Available
Direct Memory Access (DMA) Is Faster
External ADC Example: SPI and DMA
Circular Buffers
Further Reading
8. Putting Together a System
Key Matrices
Segmented Displays
Pixel Displays
Display Assets
Changeable Data? Flyweight and Factory Patterns
External Flash Memory
Display Assets
Emulated EEPROMs and KV Stores
Little File Systems
Data Storage
Analog Signals
Digital Sensors
Data Handling
Changing Algorithms: Strategy
Algorithm Stages: Pipelines and Filters
Calculating Needs: Speeds and Feeds
Data Bandwidth
Memory Throughput and Buffering
Further Reading
9. Getting into Trouble
Fighting with the Compiler Optimizations
Impossible Bugs
Reproduce the Bug
Explain the Bug
Creating Chaos and Hard Faults
Dividing by Zero
Talking to Things That Aren’t There
Running Undefined Instructions
Incorrect Memory Access (Unaligned Access)
Returning a Pointer to Stack Memory
Stack Overflows and Buffer Overflows
Debugging Hard Faults
Processor Registers: What Went Wrong?
Creating a Core Dump
Using the Core Dump
Merely Very Difficult Bugs
Consequences of Being Clever
Further Reading
10. Building Connected Devices
Connecting Remotely
Directly: Ethernet and WiFi
Through a Gateway
Via a Mesh
Robust Communication
Version!
Checksums, CRCs, Hashes
Encryption and Authentication
Risk Analysis
Updating Code
Firmware Update Security
Multiple Pieces of Code
Fallback Lifeboat
Staged Rollout
Managing Large Systems
Manufacturing
Further Reading
11. Doing More with Less
Need More Code Space
Reading a Map File (Part 1)
Process of Elimination
Libraries
Functions Versus Macros: Which Are Smaller?
Constants and Strings
Need More RAM
Remove malloc
Reading a Map File (Part 2)
Registers and Local Variables
Function Chains
Pros and Cons of Globals: RAM Versus Stack
Clever Memory Overlays
Need More Speed
Profiling
Optimizing for Processor Cycles
Summary
Further Reading
12. Math
Identifying Fast and Slow Operations
Taking an Average
Different Averages: Cumulative and Median
Using an Existing Algorithm
Designing and Modifying Algorithms
Factor Polynomials
Taylor Series
Dividing by a Constant
Scaling the Input
Lookup Tables
Fake Floating-Point Numbers
Rational Numbers
Precision
Addition (and Subtraction)
Multiplication (and Division)
Machine Learning
Look Up the Answer!
Further Reading
13. Reducing Power Consumption
Understanding Power Consumption
Measuring Power Consumption
Designing for Lower Power Consumption
Turn Off the Light When You Leave the Room
Turn Off Peripherals
Turn Off Unused I/O Devices
Turn Off Processor Subsystems
Slow Down to Conserve Energy
Putting the Processor to Sleep
Interrupt-Based Code Flow Model
A Closer Look at the Main Loop
Processor Watchdog
Avoid Frequent Wake-Ups
Chained Processors
Further Reading
14. Motors and Movement
Creating Movement
Position Encoding
Driving a Simple DC Motor with PWM
Motor Control
PID Control
Motion Profiles
Ten Things I Hate About Motors
Further Reading
Index
About the Author
Anderen die dit boek kochten, kochten ook
Rubrieken
- advisering
- algemeen management
- coaching en trainen
- communicatie en media
- economie
- financieel management
- inkoop en logistiek
- internet en social media
- it-management / ict
- juridisch
- leiderschap
- marketing
- mens en maatschappij
- non-profit
- ondernemen
- organisatiekunde
- personal finance
- personeelsmanagement
- persoonlijke effectiviteit
- projectmanagement
- psychologie
- reclame en verkoop
- strategisch management
- verandermanagement
- werk en loopbaan